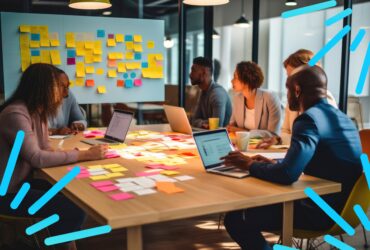
Insights
When developing a search strategy, deciding where to invest time and money can prove challenging. Should you invest more into strengthening your organic SEO presence? Or is it better to focus on bidding for paid traffic from pay-per-click (PPC) keywords with the highest conversion rates?
As you might expect, the answer is more complex than prioritising one over the other. The most effective search strategy takes the strengths of both SEO and PPC to ensure that one channel picks up where the other falls short.
This article examines how PPC and SEO can work together and develop a cohesive search strategy, which will improve SERP results and maximise ROI.
PPC is a form of digital advertising that lets businesses display ads in SERPs by paying for the real estate. In PPC, companies only pay when a user clicks their ads. These ads typically appear at the top and bottom of SERPs and are often the first results users see. Like organic SEO, Paid Search has strengths and weaknesses:
Organic SEO involves optimising a website to improve its visibility in search engine results pages (SERPs). Several factors contribute to SEO, including content, structure, site speed, and metadata. Ranking for top organic keywords can drive considerable traffic to webpages. But there are drawbacks to just relying on SEO to bring organic traffic to your website. Some pros and cons include:
As you can see, SEO and PPC have their strengths. For instance, while PPC requires constant curation, digital marketers can pivot their paid strategy quickly to try new approaches. Alternatively, SEO can give your business top-ranking results for free. But since it takes longer to rank organically than it does with PPC, if a strategy falls short and requires adjustments, this can delay the process further.
Luckily, both channels provide support in areas where the other falls short. That is why it is essential to use SEO and PPC, so you can maximise your marketing ROI and unify your search strategy. For ideas on how to do so, here’s four ways to combine organic SEO and PPC for an optimised search strategy.
A harmonious search strategy uses organic and paid search insights to inform the other. For example, suppose you find that organic traffic originating from a specific geographic area has a higher conversion rate. In that case, your PPC team can aggressively adjust bids to target users in that location. Alternatively, you can understand which keywords, calls-to-actions (CTAs), and messaging are more effective for PPC quicker than with organic. You can use these insights to target popular keywords in metadata, blogs, and landing page copy to increase organic CTR and conversions.
With PPC, the higher your bid on keywords, the lower your overall ROI. Therefore, if you can organically capture top spots for those keywords, you don’t need to spend money on them for PPC. Of course, specific keywords are highly competitive to rank organically, so if you are struggling to rank in a top position, the higher cost of PPC may be worth it. It’s all about finding the right balance.
For broad keywords with high search volumes that are difficult to rank for organically, bidding on these ensures you are listed in front of the users searching for those terms. PPC can also be a temporary fix while you build your organic strategy, which takes longer to get off the ground. For example, if you launch a new product and still need to rank for related keywords, you can bid on those keywords while you optimise your site to rank for them organically. As your organic rankings increase, you can adjust your PPC spend accordingly.
The goal of SEO and PPC is SERP visibility over competitors. However, organically ranking for competitor-branded keywords can prove challenging. Most businesses want to refrain from supporting content on their site that talks about competitor’s products or service offerings. However, with PPC, not only can you bid on competitor-branded keywords but also create ads that offer a superior alternative to those competitors. So, when potential customers search for a competitor’s product, they’ll see your search ads, providing an option they may choose.
Overall, organic SEO and PPC are powerful tools that can maximise your ROI. By understanding the strengths and weaknesses of each channel, you can develop an integrated SEO and PPC strategy that will drive more traffic to your site, increase conversions, and outrank your competitors.
Our Future of SEO report analyses current and future concerns in the SEO landscape. The rise of generative AI, changes to ranking signals and tracking multichannel user journeys are discussed in-depth, along with actionable solutions to conquer these issues.
The result is that our report provides marketers with guidance to enhance their global search strategy by harnessing the true power of SEO.
Insights
Insights
Insights